-
Play Fruity Slots Online | Fruit Slots and UK Casino Gambling Fruity Slots Betting Reviews
- Introduction
- Introduction to Odd and Even Numbers in C
- Using Modulo Operator to Check for Odd or Even
- Using Bitwise AND Operator to Check for Odd or Even
- Using Ternary Operator to Check for Odd or Even
- Using Switch Statement to Check for Odd or Even
- Using If-Else Statement to Check for Odd or Even
- Using Conditional Operator to Check for Odd or Even
- Checking Multiple Numbers for Odd or Even
- Converting Decimal Numbers to Binary and Checking for Odd or Even
- Checking Floating-Point Numbers for Odd or Even
- Using Functions to Check for Odd or Even
- Common Mistakes to Avoid When Checking for Odd or Even
- Applications of Odd and Even Numbers in C Programming
- Conclusion and Further Learning Resources
- Q&A
- Conclusion
“Effortlessly decide odd and even numbers in C programming language.”
Introduction
In C programming language, it is essential to be capable of decide whether or not a given number is odd and even. This is a fundamental operation that is usually required in numerous programming duties. In this article, we’ll talk about the right way to check if a number is odd and even in C.
Introduction to Odd and Even Numbers in C
Are you new to programming in C and questioning the right way to check if a number is odd and even? Don’t be concerned, it is a widespread query for learners. In this article, we’ll introduce you to the idea of wierd and even numbers in C and show you the right way to decide whether or not a number is odd and even.
First, let’s outline what odd and even numbers are. A good number is any integer that can be divided by 2 with out leaving a the rest. For instance, 2, 4, 6, 8, and 10 are all even numbers. On the other hand, an odd number is any integer that can not be divided by 2 with out leaving a the rest. Examples of wierd numbers include 1, 3, 5, 7, and 9.
Now that we all know what odd and even numbers are, let’s transfer on to the right way to check if a number is odd and even in C. The best manner to do that is through the use of the modulo operator (%). The modulo operator returns the rest of a division operation. For instance, 5 % 2 would return 1 as a result of 5 divided by 2 leaves a the rest of 1.
To check if a number is odd and even utilizing the modulo operator, we merely have to divide the number by 2 and check if the rest is equal to 0. If the rest is 0, then the number is even. If the rest is 1, then the number is odd.
Let’s check out some code examples to make this clearer. Here is the right way to check if a number is even in C:
“`
int num = 4;
if (num % 2 == 0) {
printf(“The number is even”);
}
“`
In this instance, we have assigned the worth of 4 to the variable num. We then use an if assertion to check if num % 2 is equal to 0. If it is, we print out “The number is even”.
Now let’s check out the right way to check if a number is odd in C:
“`
int num = 5;
if (num % 2 == 1) {
printf(“The number is odd”);
}
“`
In this instance, we have assigned the worth of 5 to the variable num. We then use an if assertion to check if num % 2 is equal to 1. If it is, we print out “The number is odd”.
It is essential to notice that the modulo operator solely works with integers. If you try to make use of it with floating-level numbers, you will get a compiler error. If you should check if a floating-level number is odd and even, you will have to convert it to an integer first.
In conclusion, checking if a number is odd and even in C is a easy process that can be completed utilizing the modulo operator. Do not forget that even numbers are divisible by 2 with out leaving a the rest, whereas odd numbers will not be. With this information, you can simply write code to check if a number is odd and even. Hold training and you may quickly change into a professional at programming in C!
Utilizing Modulo Operator to Check for Odd or Even
Are you new to programming and questioning the right way to check if a number is odd and even in C? Don’t be concerned, it is a easy process that can be completed utilizing the modulo operator.
The modulo operator, represented by the percent signal (%), returns the rest of a division operation. For instance, 5 % 2 would return 1 as a result of 2 goes into 5 twice with a the rest of 1.
To check if a number is odd and even, we can use the modulo operator to divide the number by 2. If the rest is 0, then the number is even. If the rest is 1, then the number is odd.
Let’s check out some code examples to see how this works in follow.
First, we’ll declare a variable known as “num” and assign it a worth of seven:
int num = 7;
To check if this number is odd and even, we’ll use the modulo operator:
if (num % 2 == 0) {
printf(“The number is even.”);
} else {
printf(“The number is odd.”);
}
In this instance, we’re utilizing an if-else assertion to print out whether or not the number is even or odd. The situation contained in the if assertion checks if the rest of num divided by 2 is equal to 0. If it is, then we all know that num is even and we print out “The number is even.” If the rest is not equal to 0, then we all know that num is odd and we print out “The number is odd.”
Let’s try one other instance. This time, we’ll declare a variable known as “enter” and ask the consumer to enter a number:
int enter;
printf(“Enter a number: “);
scanf(“%d”, &enter);
Now that now we have the consumer’s enter, we can check if it is odd and even utilizing the modulo operator:
if (enter % 2 == 0) {
printf(“The number is even.”);
} else {
printf(“The number is odd.”);
}
Once more, we’re utilizing an if-else assertion to print out whether or not the number is even or odd. The situation contained in the if assertion checks if the rest of enter divided by 2 is equal to 0. If it is, then we all know that enter is even and we print out “The number is even.” If the rest is not equal to 0, then we all know that enter is odd and we print out “The number is odd.”
As you can see, checking if a number is odd and even in C is a easy process that can be completed utilizing the modulo operator. Whether or not you are a newbie or an skilled programmer, it is all the time essential to have a stable understanding of the fundamentals. By mastering easy duties like this, you will be properly in your option to turning into a proficient C programmer.
Utilizing Bitwise AND Operator to Check for Odd or Even
If you are new to programming, you may be questioning the right way to check if a number is odd and even in C. Fortunately, there are a number of ways to do that, and one of the vital environment friendly strategies is through the use of the bitwise AND operator.
Earlier than we dive into the code, let’s first perceive what the bitwise AND operator does. In C, the bitwise AND operator is represented by the ampersand image (&). Once you use this operator, it compares the binary illustration of two numbers and returns a brand new number the place every bit is set to 1 provided that each corresponding bits in the unique numbers are additionally 1.
Now, let’s apply this information to checking if a number is odd and even. In binary, odd numbers all the time finish in 1, whereas even numbers all the time finish in 0. Due to this fact, if we use the bitwise AND operator to match a number with 1, we can decide if it is odd and even.
Here is the code:
“`
if (num & 1) {
printf(“The number is odd.”);
} else {
printf(“The number is even.”);
}
“`
Let’s break down what’s taking place here. The `if` assertion checks if the results of `num & 1` is true or false. If it is true, meaning the final little bit of `num` is 1, indicating that it is an odd number. If it is false, meaning the final little bit of `num` is 0, indicating that it is a fair number.
It is essential to notice that this methodology solely works for integers. If you try to make use of it with floating-level numbers, you will get a compiler error. Moreover, if you happen to try to make use of it with damaging numbers, you may get sudden outcomes. This is as a result of the bitwise AND operator works with the binary illustration of numbers, and damaging numbers are represented in two’s complement kind.
To deal with damaging numbers, you can use the modulus operator (%). Here is an instance:
“`
if (num % 2 == 0) {
printf(“The number is even.”);
} else {
printf(“The number is odd.”);
}
“`
This code checks if `num` is divisible by 2. If it is, meaning it is a fair number. If it isn’t, meaning it is an odd number.
In conclusion, checking if a number is odd and even in C is a easy process that can be completed utilizing the bitwise AND operator or the modulus operator. By understanding how these operators work, you can write environment friendly and correct code that can help you in your programming journey. Hold training and do not be afraid to experiment with completely different strategies!
Utilizing Ternary Operator to Check for Odd or Even
In programming, it is usually essential to find out whether or not a given number is odd and even. This can be helpful in a wide range of purposes, from easy mathematical calculations to more advanced algorithms. In C, there are a number of ways to check if a number is odd and even, however one of the vital environment friendly and concise strategies is to make use of the ternary operator.
The ternary operator is a shorthand manner of writing an if-else assertion. It takes three operands: a situation, a worth to return if the situation is true, and a worth to return if the situation is false. The syntax of the ternary operator is as follows:
situation ? value_if_true : value_if_false;
To make use of the ternary operator to check if a number is odd and even, we can use the modulus operator (%), which returns the rest of a division operation. If a number is even, it is going to don’t have any the rest when divided by 2, whereas an odd number may have a the rest of 1.
Here is an instance of the right way to use the ternary operator to check if a number is odd and even in C:
int num = 7;
char* end result = (num % 2 == 0) ? “even” : “odd”;
printf(“%d is %sn”, num, end result);
In this instance, we declare a variable num and assign it the worth 7. We then use the ternary operator to check if num is even or odd by checking if num % 2 is equal to 0. If it is, we return the string “even”, in any other case we return the string “odd”. We then print out the end result utilizing printf.
This code will output the next:
7 is odd
As you can see, the ternary operator permits us to check if a number is odd and even in only one line of code. This can be particularly helpful in conditions the place we have to carry out this check a number of times, or the place we have to write code that is as concise and environment friendly as potential.
After all, there are other ways to check if a number is odd and even in C. One widespread methodology is to make use of an if-else assertion:
int num = 7;
if (num % 2 == 0) {
printf(“%d is evenn”, num);
} else {
printf(“%d is oddn”, num);
}
This code will output the identical end result because the earlier instance:
7 is odd
Whereas this code is completely legitimate, it is longer and fewer concise than the ternary operator model. In addition, if we have to carry out this check a number of times in our code, utilizing the ternary operator can help to cut back code duplication and make our code simpler to read and preserve.
In conclusion, the ternary operator is a strong instrument in C that can be used to check if a number is odd and even in only one line of code. Whereas there are other ways to carry out this check, utilizing the ternary operator can help to make our code more concise and environment friendly. So the subsequent time you should check if a number is odd and even in C, think about using the ternary operator and see the way it can simplify your code.
Utilizing Swap Assertion to Check for Odd or Even
In programming, it is usually essential to find out whether or not a given number is odd and even. This can be helpful in a wide range of purposes, from easy mathematical calculations to more advanced algorithms. In C, there are a number of ways to check whether or not a number is odd and even, however one of the vital environment friendly and simple strategies is to make use of a change assertion.
A change assertion is a management construction that lets you take a look at a variable in opposition to a collection of values and execute completely different code blocks relying on the end result. In the case of checking for odd and even numbers, we can use a change assertion to check the rest of the number when divided by 2. If the rest is 0, the number is even; if the rest is 1, the number is odd.
To make use of a change assertion to check for odd and even numbers in C, we first have to declare a variable to carry the number we wish to take a look at. This can be any integer worth, equivalent to:
int num = 42;
Subsequent, we have to use the modulo operator (%) to calculate the rest of the number when divided by 2. This can be performed utilizing the next code:
int the rest = num % 2;
The modulo operator returns the rest of a division operation, so if num is even, the rest can be 0; if num is odd, the rest can be 1.
Now that now we have the rest worth, we can use a change assertion to check it and execute completely different code blocks relying on whether or not the number is odd and even. Here is an instance:
change (the rest) {
case 0:
printf(“%d is even.”, num);
break;
case 1:
printf(“%d is odd.”, num);
break;
}
In this code, we’re utilizing the change assertion to check the worth of the rest. If the rest is 0, we print a message indicating that the number is even. If the rest is 1, we print a message indicating that the number is odd. The break assertion is used to exit the change assertion as soon as the proper code block has been executed.
Utilizing a change assertion to check for odd and even numbers in C is a easy and environment friendly option to carry out this widespread process. It lets you take a look at a variable in opposition to a collection of values and execute completely different code blocks relying on the end result, making it a strong instrument for a lot of programming purposes.
If you are new to programming or simply getting began with C, utilizing a change assertion to check for odd and even numbers is a good way to follow your abilities and learn more about how management constructions work. With a bit follow, you can use this method to unravel a variety of programming issues and construct more advanced algorithms. So why not give it a try at present?
Utilizing If-Else Assertion to Check for Odd or Even
If you’re new to programming, you may be questioning the right way to check if a number is odd and even in C. Thankfully, it is a easy process that can be completed utilizing an if-else assertion.
First, let’s outline what we imply by odd and even numbers. A good number is any integer that can be divided by 2 with out leaving a the rest. Examples of even numbers include 2, 4, 6, 8, and so forth. On the other hand, an odd number is any integer that can not be divided by 2 with out leaving a the rest. Examples of wierd numbers include 1, 3, 5, 7, and so forth.
To check if a number is odd and even in C, we can use the modulo operator (%). The modulo operator returns the rest of a division operation. For instance, 5 % 2 would return 1 as a result of 5 divided by 2 leaves a the rest of 1.
Now, let’s check out the code to check if a number is odd and even in C:
“`
#include
int principal() {
int num;
printf(“Enter an integer: “);
scanf(“%d”, &num);
if (num % 2 == 0) {
printf(“%d is even.”, num);
}
else {
printf(“%d is odd.”, num);
}
return 0;
}
“`
Let’s break down the code. First, we declare an integer variable known as num. We then immediate the consumer to enter an integer utilizing the printf and scanf capabilities. The consumer’s enter is saved in the num variable.
Subsequent, we use an if-else assertion to check if the num variable is even or odd. If the num variable is divisible by 2 (i.e., num % 2 == 0), we print out a message saying that the number is even. In any other case, we print out a message saying that the number is odd.
It is essential to notice that the if-else assertion is enclosed in curly braces ({}) to point the block of code that needs to be executed if the situation is true or false. Additionally, the equality operator (==) is used to match the results of the modulo operation to 0.
Now that you understand how to check if a number is odd and even in C, you can use this information to write down more advanced applications. For instance, you may write a program that prompts the consumer to enter a collection of integers after which calculates the sum of the even numbers.
In conclusion, checking if a number is odd and even in C is a easy process that can be completed utilizing an if-else assertion and the modulo operator. With this information, you can write more advanced applications that contain even and odd numbers. So, do not be afraid to experiment and have fun together with your code!
Utilizing Conditional Operator to Check for Odd or Even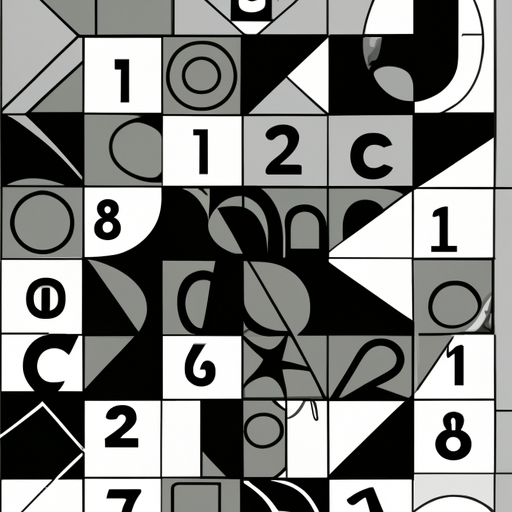
If you’re new to programming, you may be questioning the right way to check if a number is odd and even in C. Thankfully, it is a easy process that can be completed utilizing conditional operators.
First, let’s outline what we imply by odd and even numbers. A good number is any integer that can be divided by 2 with out leaving a the rest. Examples of even numbers include 2, 4, 6, 8, and so forth. On the other hand, an odd number is any integer that can not be divided by 2 with out leaving a the rest. Examples of wierd numbers include 1, 3, 5, 7, and so forth.
To check if a number is odd and even in C, we can use the modulo operator (%). The modulo operator returns the rest of a division operation. For instance, 5 % 2 would return 1 as a result of 5 divided by 2 leaves a the rest of 1.
To check if a number is even, we can use the modulo operator to check if the rest of the number divided by 2 is equal to 0. If the rest is 0, then the number is even. Here is an instance:
“`
int num = 4;
if (num % 2 == 0) {
printf(“The number is even”);
}
“`
In this instance, we declare a variable known as num and assign it the worth of 4. We then use an if assertion to check if the rest of num divided by 2 is equal to 0. If it is, we print out a message saying that the number is even.
To check if a number is odd, we can use the identical strategy however with a slight modification. Instead of checking if the rest of the number divided by 2 is equal to 0, we check if it is equal to 1. Here is an instance:
“`
int num = 5;
if (num % 2 == 1) {
printf(“The number is odd”);
}
“`
In this instance, we declare a variable known as num and assign it the worth of 5. We then use an if assertion to check if the rest of num divided by 2 is equal to 1. If it is, we print out a message saying that the number is odd.
It is essential to notice that the modulo operator can additionally return damaging remainders. For instance, -5 % 2 would return -1 as a result of -5 divided by 2 leaves a the rest of -1. To deal with damaging remainders, we can add 2 to the results of the modulo operation if it is damaging. Here is an instance:
“`
int num = -5;
if (num % 2 == -1 || num % 2 == 1) {
printf(“The number is odd”);
} else {
printf(“The number is even”);
}
“`
In this instance, we declare a variable known as num and assign it the worth of -5. We then use an if assertion to check if the rest of num divided by 2 is equal to -1 or 1. If it is, we print out a message saying that the number
Checking A number of Numbers for Odd or Even
In programming, it is usually essential to check whether or not a number is odd and even. This is a easy process that can be completed utilizing the modulus operator in C. Nonetheless, what if you should check a number of numbers for odd and even? In this article, we’ll discover completely different ways to check a number of numbers for odd and even in C.
One option to check a number of numbers for odd and even is to make use of a loop. A loop is a programming assemble that lets you repeat a set of directions a number of times. In C, there are several types of loops, such because the for loop, the whereas loop, and the do-whereas loop. For this instance, we’ll use the for loop.
To make use of a for loop to check a number of numbers for odd and even, you first have to outline an array to retailer the numbers. An array is a group of variables of the identical type. In this case, we’ll outline an array of integers.
int numbers[5] = {1, 2, 3, 4, 5};
Subsequent, you should use a for loop to iterate by way of the array and check every number for odd and even. To check if a number is odd and even, you can use the modulus operator (%). The modulus operator returns the rest of a division operation. If a number is even, the rest when divided by 2 can be 0. If a number is odd, the rest when divided by 2 can be 1.
for (int i = 0; i < 5; i++) {
if (numbers[i] % 2 == 0) {
printf("%d is evenn", numbers[i]);
} else {
printf("%d is oddn", numbers[i]);
}
}
In this instance, we use a for loop to iterate by way of the array of numbers. For every number, we check if it is even or odd utilizing the modulus operator. If the rest is 0, we print a message indicating that the number is even. If the rest is 1, we print a message indicating that the number is odd.
One other option to check a number of numbers for odd and even is to make use of a operate. A operate is a block of code that performs a selected process. In C, you can outline your personal capabilities to carry out customized duties. To check if a number is odd and even utilizing a operate, you can outline a operate that takes an integer as enter and returns a string indicating whether or not the number is odd and even.
char* check_odd_even(int number) {
if (number % 2 == 0) {
return "even";
} else {
return "odd";
}
}
In this instance, we outline a operate known as check_odd_even that takes an integer as enter and returns a string. Inaspect the operate, we use the modulus operator to check if the number is even or odd. If the rest is 0, we return the string "even". If the rest is 1, we return the string "odd".
To make use of this operate to check a number of numbers for odd and even, you can outline an array of integers and iterate by way of the array, calling the operate for every number.
int
Changing Decimal Numbers to Binary and Checking for Odd or Even
If you are new to programming, you may be questioning the right way to check if a number is odd and even in C. Thankfully, it is a easy course of that can be completed with just some traces of code.
Earlier than we dive into the code, let’s first review what it means for a number to be odd and even. A good number is any integer that can be divided by 2 with out leaving a the rest. Examples of even numbers include 2, 4, 6, 8, and so forth. On the other hand, an odd number is any integer that can not be divided by 2 with out leaving a the rest. Examples of wierd numbers include 1, 3, 5, 7, and so forth.
Now that we perceive the distinction between odd and even numbers, let’s transfer on to the code. Step one is to transform the decimal number to binary. This is essential as a result of we have to look in any case vital bit (LSB) of the binary illustration to find out if the number is odd and even.
To transform a decimal number to binary in C, we can use the bitwise AND operator (&) and the proper shift operator (>>). Here is an instance:
int decimal_number = 10;
int binary_number = decimal_number & 1;
In this instance, we’re changing the decimal number 10 to binary. The bitwise AND operator (&) is used to match the decimal number to the binary number 1. The results of this comparability is saved in the variable binary_number. If the decimal number is even, the LSB of the binary illustration can be 0. If the decimal number is odd, the LSB of the binary illustration can be 1.
Subsequent, we have to check the worth of binary_number to find out if the decimal number is odd and even. We can do that utilizing an if assertion:
if (binary_number == 0) {
printf(“The number is even.”);
} else {
printf(“The number is odd.”);
}
In this instance, we’re utilizing an if assertion to check if binary_number is equal to 0. If it is, we print the message “The number is even.” If it isn’t, we print the message “The number is odd.”
It is essential to notice that this methodology solely works for integers. If you are working with floating-level numbers, you will want to make use of a special strategy.
In conclusion, checking if a number is odd and even in C is a easy course of that can be completed with just some traces of code. By changing the decimal number to binary and looking out on the LSB, we can decide if the number is odd and even. With a bit follow, you can check for odd and even numbers in no time!
Checking Floating-Level Numbers for Odd or Even
Relating to programming, one of the vital fundamental duties is checking whether or not a number is odd and even. This may appear to be a easy process, however it can be a bit tough when coping with floating-level numbers. In this article, we’ll discover the right way to check if a number is odd and even in C, particularly when coping with floating-level numbers.
Firstly, it is essential to grasp what floating-level numbers are. Floating-level numbers are numbers which have a decimal level in them. They’re represented in a pc’s reminiscence utilizing a selected format known as the IEEE 754 commonplace. This format permits for a variety of numbers to be represented, together with very small and really giant numbers.
Relating to checking whether or not a floating-level number is odd and even, we have to first convert the number to an integer. This is as a result of the idea of wierd and even solely applies to integers. To do that, we can use the ground() operate, which rounds a floating-level number all the way down to the closest integer.
As soon as now we have the integer worth of the floating-level number, we can then check if it is odd and even utilizing the modulus operator (%). The modulus operator returns the rest of a division operation. If the rest is 0, then the number is even. If the rest is 1, then the number is odd.
Let’s check out some code that demonstrates this:
“`
#include
#include
int principal() {
float num = 3.14;
int intNum = flooring(num);
if (intNum % 2 == 0) {
printf(“%f is evenn”, num);
} else {
printf(“%f is oddn”, num);
}
return 0;
}
“`
In this instance, now we have a floating-level number `num` with a worth of three.14. We first use the `flooring()` operate to transform it to an integer, which provides us a worth of three. We then use the modulus operator to check if it is odd and even. Since 3 % 2 is equal to 1, we print out that the number is odd.
It is essential to notice that when coping with floating-level numbers, there can be some precision points. Which means the integer worth we get from utilizing the `flooring()` operate may not be precisely what we count on. For instance, if now we have a floating-level number with a worth of three.999999, the `flooring()` operate will spherical it down to three, despite the fact that we may count on it to be 4. This can result in incorrect outcomes when checking if a number is odd and even.
To avoid this problem, we can add a small epsilon worth to the floating-level number earlier than changing it to an integer. This epsilon worth is a really small number that is added to the floating-level number to ensure that it is rounded as much as the closest integer. Right here is an instance:
“`
#include
#include
int principal() {
float num = 3.999999;
int intNum = flooring(num + 0.000001);
if (intNum
Utilizing Capabilities to Check for Odd or Even
Are you new to programming and questioning the right way to check if a number is odd and even in C? Don’t be concerned, it is a widespread query for learners. In this article, we’ll discover the right way to use capabilities to check for odd and even numbers in C.
First, let’s outline what odd and even numbers are. A good number is any integer that can be divided by 2 with out leaving a the rest. For instance, 2, 4, 6, and eight are even numbers. On the other hand, an odd number is any integer that can not be divided by 2 with out leaving a the rest. For instance, 1, 3, 5, and seven are odd numbers.
Now that we all know what odd and even numbers are, let’s dive into the right way to check for them in C. One option to check if a number is odd and even is to make use of the modulus operator (%). The modulus operator returns the rest of a division operation. If a number is even, then dividing it by 2 will end result in a the rest of 0. If a number is odd, then dividing it by 2 will end result in a the rest of 1.
Here is an instance of utilizing the modulus operator to check if a number is odd and even:
“`
#include
int principal() {
int num;
printf(“Enter an integer: “);
scanf(“%d”, &num);
if (num % 2 == 0) {
printf(“%d is even.”, num);
}
else {
printf(“%d is odd.”, num);
}
return 0;
}
“`
In this instance, we first immediate the consumer to enter an integer utilizing the `printf` and `scanf` capabilities. We then use an `if` assertion to check if the number is even or odd utilizing the modulus operator. If the rest of `num` divided by 2 is 0, then we all know that `num` is even and we print a message saying so. In any other case, we all know that `num` is odd and we print a message saying that as a substitute.
Whereas this methodology works, it can be tedious to write down the identical code time and again each time you should check if a number is odd and even. That is the place capabilities come in useful. Capabilities can help you encapsulate a block of code that performs a selected process and reuse it all through your program.
Here is an instance of a operate that checks if a number is odd and even:
“`
#include
int isEven(int num) {
if (num % 2 == 0) {
return 1;
}
else {
return 0;
}
}
int principal() {
int num;
printf(“Enter an integer: “);
scanf(“%d”, &num);
if (isEven(num)) {
printf(“%d is even.”, num);
}
else {
printf(“%d is odd.”, num);
}
return 0;
}
“`
In this instance, we outline a operate known as `isEven` that takes an integer parameter `num`. The operate checks if `num` is even utilizing
Frequent Errors to Avoid When Checking for Odd or Even
Relating to programming, checking whether or not a number is odd and even is a typical process. It may appear to be a easy process, however there are some widespread errors that programmers make when checking for odd and even numbers in C. In this article, we’ll talk about a few of these errors and the right way to avoid them.
Probably the most widespread errors that programmers make when checking for odd and even numbers is utilizing the flawed operator. The modulus operator (%) is used to find the rest of a division operation. When checking for odd and even numbers, the modulus operator is used to find out whether or not a number is divisible by 2. If the rest is 0, then the number is even. If the rest is 1, then the number is odd. Nonetheless, some programmers mistakenly use the division operator (/) as a substitute of the modulus operator. This can result in incorrect outcomes and sudden habits in this system.
One other mistake that programmers make when checking for odd and even numbers is not contemplating damaging numbers. When a damaging number is divided by 2, the rest can be both -1 or 1. Which means a damaging number can be each odd and even. To avoid this error, programmers ought to use absolutely the worth operate (abs()) to transform damaging numbers to constructive numbers earlier than checking for odd and even.
Programmers may additionally make the error of utilizing floating-level numbers as a substitute of integers when checking for odd and even numbers. Floating-level numbers will not be exact and can result in rounding errors. This can trigger sudden habits in this system and result in incorrect outcomes. To avoid this error, programmers ought to use integers when checking for odd and even numbers.
One other widespread mistake that programmers make when checking for odd and even numbers is not utilizing parentheses accurately. When utilizing the modulus operator, it is essential to make use of parentheses to group the operation accurately. For instance, if a programmer needs to check whether or not a number is odd and even, they need to use the next code:
if ((num % 2) == 0) {
printf(“Even”);
} else {
printf(“Odd”);
}
Utilizing parentheses accurately ensures that the modulus operation is carried out earlier than the comparability operation.
In conclusion, checking for odd and even numbers in C may appear to be a easy process, however there are some widespread errors that programmers make. These errors include utilizing the flawed operator, not contemplating damaging numbers, utilizing floating-level numbers as a substitute of integers, and never utilizing parentheses accurately. By avoiding these errors, programmers can ensure that their applications work accurately and produce the anticipated outcomes. So, subsequent time you should check whether or not a number is odd and even in C, keep in mind to make use of the modulus operator, think about damaging numbers, use integers, and use parentheses accurately. Comfortable coding!
Functions of Odd and Even Numbers in C Programming
C programming is a strong instrument that can be used to unravel a variety of issues. Probably the most fundamental duties that you just can carry out in C is to check whether or not a number is odd and even. This may appear to be a trivial process, however it has many sensible purposes in programming.
To check whether or not a number is odd and even in C, you should use the modulus operator (%). This operator returns the rest of a division operation. If the rest is zero, then the number is even. If the rest is one, then the number is odd.
For instance, as an instance you wish to check whether or not the number 7 is odd and even. You can use the next code:
“`
if (7 % 2 == 0) {
printf(“7 is even”);
} else {
printf(“7 is odd”);
}
“`
In this code, we use the modulus operator to divide 7 by 2. The rest is 1, so we all know that 7 is odd. We then use an if-else assertion to print out the suitable message.
This may appear to be a easy instance, however there are numerous sensible purposes for checking whether or not a number is odd and even in C programming. For instance, you may use this method to carry out completely different operations on odd and even numbers.
For example you wish to write a program that calculates the sum of all even numbers between 1 and 100. You can use the next code:
“`
int sum = 0;
for (int i = 1; i <= 100; i++) {
if (i % 2 == 0) {
sum += i;
}
}
printf("The sum of all even numbers between 1 and 100 is %d", sum);
“`
In this code, we use a for loop to iterate by way of all numbers between 1 and 100. We then use the modulus operator to check whether or not every number is even. If it is, we add it to the sum variable. Lastly, we print out the sum.
This is only one instance of the way you can use odd and even numbers in C programming. There are a lot of other purposes as properly. For instance, you may use this method to carry out completely different operations on odd and even indices in an array.
In addition to being helpful, checking whether or not a number is odd and even in C programming is additionally simple to do. All you want is the modulus operator and a fundamental understanding of the way it works.
If you're new to C programming, don't be intimidated by this idea. It's a basic a part of the language, and when you perceive it, you'll be capable of use it in many alternative ways.
To get started, try writing some easy applications that use odd and even numbers. Experiment with completely different operations and see what you can give you. With a bit follow, you'll quickly be capable of use this method to unravel all kinds of programming issues.
In conclusion, checking whether or not a number is odd and even in C programming is a easy however highly effective approach that has many sensible purposes. Whether or not you're writing a program to calculate the sum of even numbers or performing completely different operations on odd and even indices in an array, this
Conclusion and Additional Studying Sources
In conclusion, checking if a number is odd and even in C is a basic idea that each programmer ought to perceive. It is a easy process that can be completed utilizing the modulus operator, which returns the rest of a division operation. By dividing the number by 2 and checking if the rest is equal to 0, we can decide if the number is even or odd.
Nonetheless, it is essential to notice that there are other ways to check if a number is odd and even in C. As an illustration, we can use bitwise operators to check the least vital little bit of the number. If the bit is 0, the number is even, and if it is 1, the number is odd. This methodology is quicker than utilizing the modulus operator, particularly when coping with giant numbers.
Moreover, there are numerous other ideas in C programming which might be value exploring. As a newbie, it is important to be taught the fundamentals of C programming, equivalent to information varieties, variables, operators, and management constructions. After getting grasp of those ideas, you can transfer on to more superior subjects equivalent to arrays, pointers, capabilities, and constructions.
There are a lot of resources accessible online that can help you be taught C programming. Web sites equivalent to Codecademy, Udemy, and Coursera offer online programs that cowl numerous points of C programming. These programs are designed for learners and supply a step-by-step guide to studying C programming.
Moreover, there are numerous books on C programming that you just can read to enhance your abilities. A number of the fashionable books include “The C Programming Language” by Brian Kernighan and Dennis Ritchie, “C Programming Absolute Newbie’s Guide” by Greg Perry and Dean Miller, and “Head First C” by David Griffiths and Daybreak Griffiths.
In conclusion, checking if a number is odd and even in C is a easy process that each programmer ought to perceive. It is a basic idea that varieties the premise of many algorithms and applications. As a newbie, it is important to be taught the fundamentals of C programming and discover other ideas equivalent to arrays, pointers, capabilities, and constructions. There are a lot of resources accessible online and in print that can help you enhance your abilities and change into a proficient C programmer. So, continue learning and training, and you’ll quickly change into a grasp of C programming.
Q&A
1. How do you check if a number is odd and even in C?
– You can use the modulo operator (%) to check if a number is odd and even in C.
2. What is the modulo operator in C?
– The modulo operator (%) returns the rest of a division operation.
3. How do you utilize the modulo operator to check if a number is odd and even in C?
– You can use the modulo operator with the number 2. If the end result is 0, the number is even. If the end result is 1, the number is odd.
4. Are you able to give an instance of utilizing the modulo operator to check if a number is odd and even in C?
– int num = 5;
if (num % 2 == 0) {
printf(“The number is even.”);
} else {
printf(“The number is odd.”);
}
5. What does the % operator do in C?
– The % operator returns the rest of a division operation.
6. What is a fair number?
– A good number is a number that is divisible by 2 with out leaving a the rest.
7. What is an odd number?
– An odd number is a number that is not divisible by 2 with out leaving a the rest.
8. Can you utilize the modulo operator with any number to check if it is odd and even in C?
– No, you can solely use the modulo operator with the number 2 to check if a number is odd and even in C.
9. What occurs if you happen to use the modulo operator with a damaging number in C?
– The results of the modulo operator with a damaging number in C is implementation-outlined.
10. What is implementation-outlined habits in C?
– Implementation-outlined habits in C is habits that is not specified by the C commonplace, however is left as much as the implementation to outline.
11. Can you utilize the modulo operator with a floating-level number in C?
– No, the modulo operator can solely be used with integer operands in C.
12. What is an operand in C?
– An operand in C is a worth or expression that is used in an operation.
13. What is an operator in C?
– An operator in C is a logo or key phrase that performs an operation on one or more operands.
14. What are some other ways to check if a number is odd and even in C?
– You can use bitwise operators or logical operators to check if a number is odd and even in C.
Conclusion
In conclusion, checking if a number is odd and even in C can be performed utilizing the modulus operator (%). If the rest of the number divided by 2 is 0, then the number is even. In any other case, it is odd. This straightforward logic can be used in numerous programming purposes.